Cloning GitHub repositories with Total.js
With this solution you can clone private/public repositories from GitHub to your server. I use the same solution in Total.js Membership.
- operations are supported in Total.js
+v2.4.0
First step
- install
git
terminal app
- create a directory
/your-app/github/
- then create a Personal access token on GitHub:
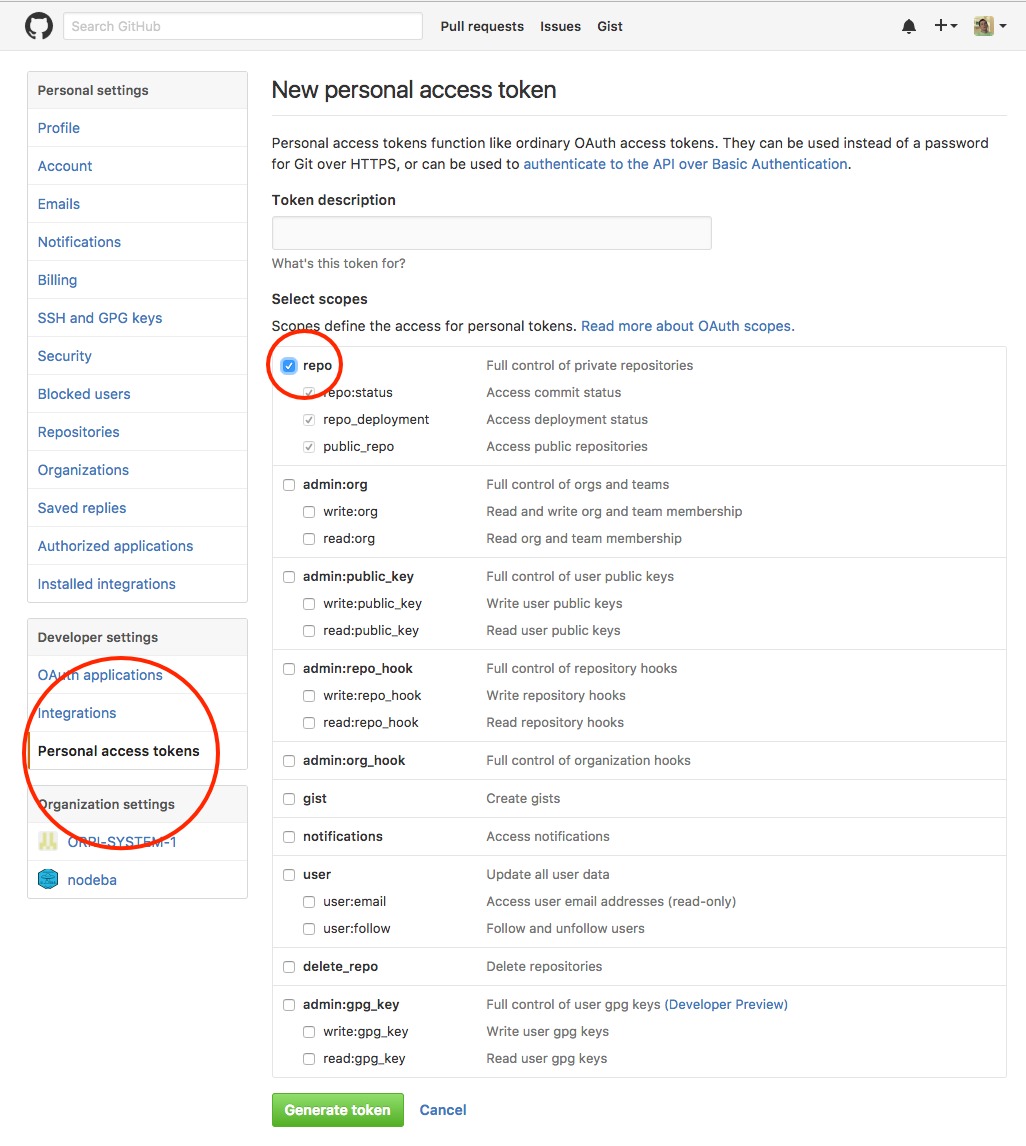
Create a bash script
We have to create a bash script which clones the repository and removes unwanted files.
/your-app/github.sh
:
# remove all files in the target directory
rm -rf $2
# clone the repo
git clone $1
# update permissions
chmod -R 777 $2
# remove unwanted files
cd $2
rm -rf .git
rm -f .gitignore
rm -f .npmignore
Create an operation
Create an operation file e.g. /your-app/definitions/operations.js
:
const Exec = require('child_process').exec;
// This operation clones GitHub repositories
NEWOPERATION('clone', function(error, value, callback) {
// value.directory
// value.url
OPTIONS.cwd = F.path.root('/github/');
Exec('bash {0} {1} {2}'.format(F.path.root('github.sh'), value.url, value.directory), OPTIONS, function(err, response) {
err && error.push(err);
callback(F.path.root('/github/' + value.directory));
});
});
How to use it?
You can execute the code below anywhere in your Total.js application:
// without the Access Token
OPERATION('clone', { directory: 'jComponent', url: 'https://github.com/totaljs/jComponent.git' }, console.log);
// with the Access Token
OPERATION('clone', { directory: 'MyRepository', url: 'https://USERNAME:TOKEN@github.com/blablabla/blablabla.git' }, console.log);